About the basics of Maven
Published on
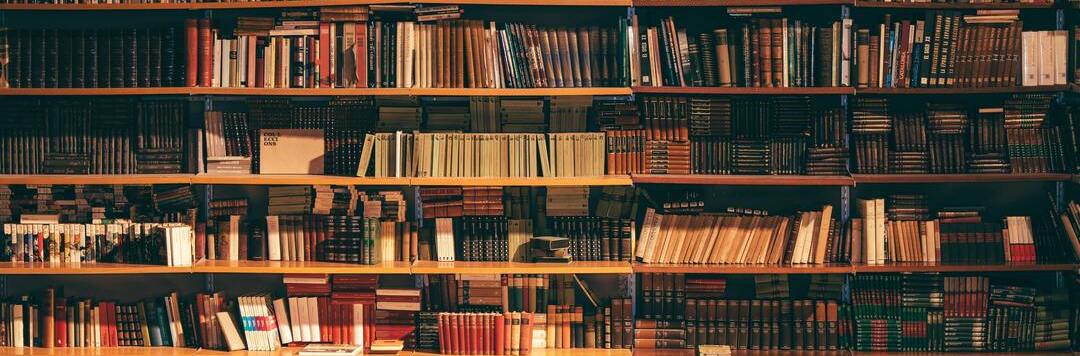
Maven is a build system and dependency manager developed by Apache. If in the past you had to write a huge cmake
file to compile all your headers and link them together, modern projects use build tools, like Maven, to automatically download dependencies, build the source code and eventually package it for deployment.
You can tell that a project uses Maven as a build system if it has a pom.xml
file in the root directory. The POM (Project Object Model) contains meta-information related to the project and build instructions.
Build lifecycles
Maven is based around the central concept of a build lifecycle. What this means is that the process for building and distributing a particular artifact (project) is clearly defined.
For the person building a project, this means that it is only necessary to learn a small set of commands to build any Maven project, and the POM will ensure they get the results they desired.
There are three built-in build lifecycles: default, clean and site.
default
lifecycle handles your project development and deployment,clean
lifecycle handles project cleaning - removes files generated at build-time in a project directory (.o
files in C or.class
files in Java),site
lifecycle handles the creation of your project's site documentation.
Lifecycle phases
Each lifecycle is composed out of phases.
Maven lifecycle cardinality
The default build lifecycle has 23 phases (see all the phases here) but the most used are:
validate
- validate the project is correct and all necessary information is available - mainly used to check if the changes in the POM work before compiling the project,compile
- compile the source code of the project,test
- test the compiled source code using a suitable unit testing framework - these tests should not require the code to be packaged or deployed,package
- take the compiled code and package it in its deployable format, such as a JAR,verify
- run any checks on results of integration tests to ensure quality criteria are met,install
- install the package into the local repository, for use as a dependency in other projects locally,deploy
- done in the build environment, copies the final package to the remote repository for sharing with other developers and projects.
Phases can be combined and executed in the mentioned order: mvn clean compile
. Notice that clean
comes from the clean lifecycle while compile
comes from the default lifecycle.
Phases are executed in a specific order. This means that if we run a specific phase using the command mvn <phase>
it won't only execute the specified phase but all the preceding phases as well.
Maven goals
Each phase is a sequence of goals, and each goal is responsible for a simple specific task. Goals have the smallest granularity in the Maven build system. When we run a phase, all goals bound to this phase are executed in order.
Maven plugins
A Maven plugin is a group of goals. However, these goals aren't necessarily all bound to the same phase. We can run a single goal with the format mvn plugin:goal
. For example, if we defined a Spring Boot plugin that has the goal run
then we can run this goal explicitly, without running the whole phase, like this: mvn spring-boot:run
.